Easiest Contact Form [Ruby/Rails example]
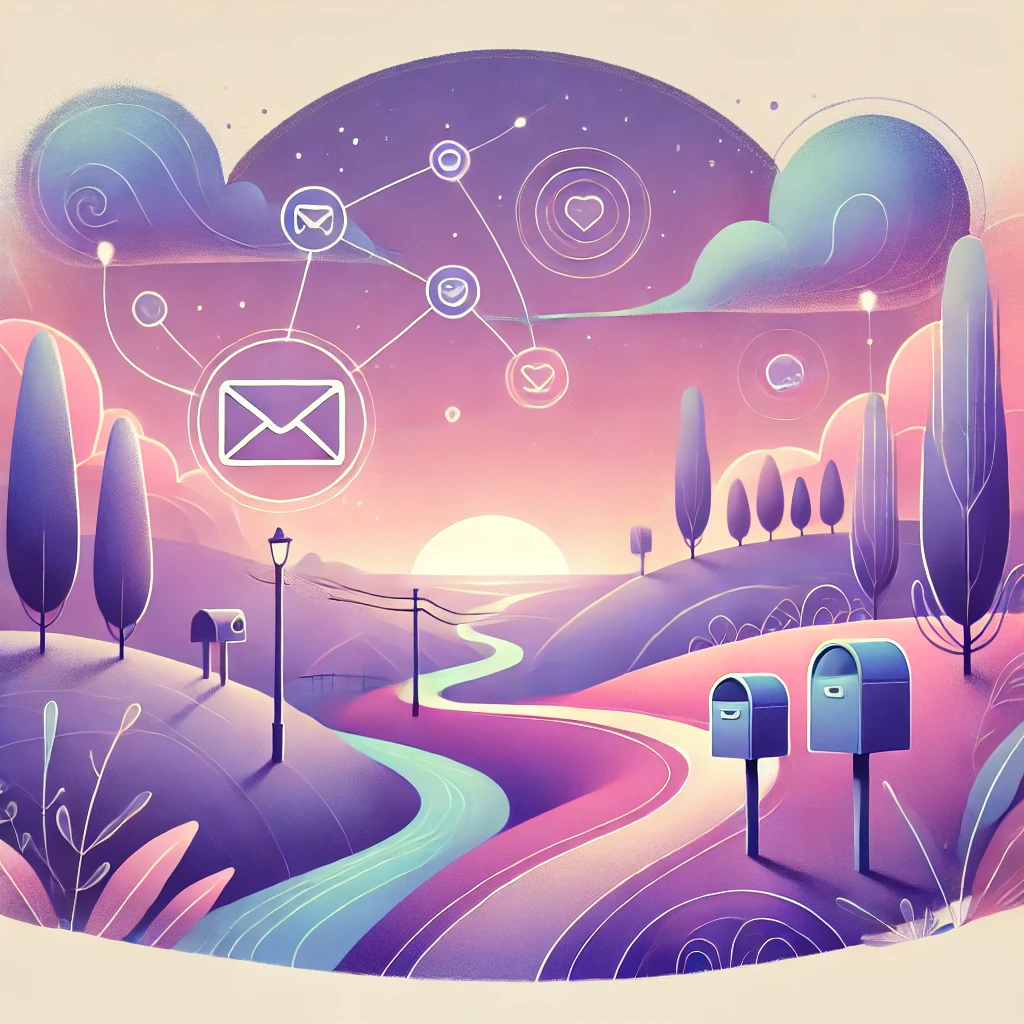
FlexLogs messaging can be used for a lot of different uses in your application, so this is an example everyone can relate to: the humble contact form. FlexLogs makes it easy to record and notify contact form submissions in your application. Whenever a user submits your contact form, you'll know instantly.
There are a million ways to handle contact form submissions, this just happens to be one of the easiest and most flexible ways to do it. Once you have FlexLogs hooked up to your application, you don't need to set up anything new for this contact form example.
With FlexLogs messaging, you can:
- Receive alerts whenever someone fills out your contact form
- Include key form fields (like Name, Email, and Message) in the notification
- Customize the channel or recipient (e.g., Slack #support or your support@ inbox)
- Keep a centralized record of all inbound requests in FlexLogs
This guide shows how to set up a basic contact form handler in Ruby (Rails-style) and leverage FlexLogs messaging to create a convenient notification workflow.
Core Messaging Format
FlexLogs recognizes special log lines that start with flexlogs
. Within those curly braces,
you specify the fields needed for your chosen notification method. The most common keys for messaging are:
Key | Description | Example | Required? |
---|---|---|---|
message | Specifies the message destination type | 'slack', 'email', or 'discord' | Yes |
label | Short tag prepended to the message (in brackets) | 'Contact Form' | No |
content | Main text body of the notification | 'New contact form from Jane Doe: Hey, I have a question...' | Yes |
channel | Slack/Discord channel to post to | '#support' | Required for Slack/Discord |
to | Email recipient(s) for the notification | 'me@example.com' | Required for Email |
tags | Optional tags for categorization within FlexLogs | ['contact', 'web'] | No |
FlexLogs parses the log line and, if it matches the format flexlogs{...}
,
parses it accordingly. Below are specific examples for Slack and email.
Slack Notification Example
If you want a Slack message posted to #support whenever someone submits
the contact form, use message: 'slack'
and specify the channel, label (optional),
and the content (the text to send).
# app/controllers/contact_controller.rb (Rails)
class ContactController < ApplicationController
def create
name = params[:name]
email = params[:email]
message_body = params[:message_body]
Rails.logger.info(
"flexlogs{
message: 'slack',
label: 'Contact Form',
content: 'Contact form submission from #{name} [#{email}]: #{message_body}',
channel: '#support'
}"
)
# ... additional logic, responding to request, etc.
render json: { success: true }, status: :ok
end
end
In this example:
-
message: 'slack'
tells FlexLogs to send a Slack notification. -
label: 'Contact Form'
will prepend "[Contact Form]" to the Slack message. -
content
includes dynamic fields (like the user's name) from the form submission. -
channel: '#support'
directs the message to the #support channel.
Email Notification Example
You can also send an email alert. Just change message
to 'email'
,
provide the to
address, and include the content.
# app/controllers/contact_controller.rb (Rails)
class ContactController < ApplicationController
def create
name = params[:name]
email = params[:email]
message_body = params[:message_body]
Rails.logger.info(
"flexlogs{
message: 'email',
label: 'Contact Form',
content: 'Contact form submission from #{name} [#{email}]: #{message_body}',
to: 'me@example.com'
}"
)
# ... additional logic, responding to request, etc.
render json: { success: true }, status: :ok
end
end
Here:
-
message: 'email'
triggers an email notification through FlexLogs. -
to: 'me@example.com'
is the recipient of the alert. -
label: 'Contact Form'
will appear in the email subject or body as "[Contact Form]". -
content
includes the details from the form submission.
Tags and Other Fields
You can also add tags
to help categorize, search,
or filter these messages later in the FlexLogs dashboard:
Rails.logger.info(
"flexlogs{
message: 'email',
label: 'Contact Form',
content: '#{name} [#{email}] says: #{message_body}',
channel: '#support',
tags: ['inbound-lead', 'contact-form', 'contact-form.home-screen']
}"
)
The tags
array doesn't affect Slack/email delivery, but
helps you organize or retrieve messages later in FlexLogs.
You can use tags to group messages by type, source, or other criteria.
Maybe you want a contact form on every page? Add a tag to track where the submission came from.
Testing and Verification
After updating your form submission code, submit a test contact form entry. Then check:
- The appropriate Slack channel or email inbox for your new notification
- Your FlexLogs events or messaging page, to see if the message was properly parsed and recorded
If you don’t see the notification, ensure that:
- You have properly set up FlexLogs and you see the log line in your live-tail page
-
The log line starts with
flexlogs{
and includes the required fields (message
,content
, etc.) - The code uses correct string quoting and concatenation
Once confirmed, you’ll have real-time alerts in your communication channels and a searchable record in FlexLogs for all contact form submissions.
Want to learn more about FlexLogs Messaging?
Check out the
Messaging documentation
for advanced options
like custom Slack attachments, webhook usage, or additional message formatting.